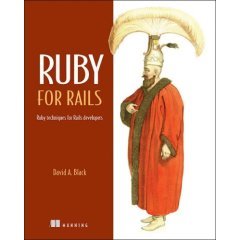
Ruby for Rails
Ruby techniques for Rails developers by David A. Black
Ruby for Rails helps Rails developers achieve Ruby mastery. Each chapter deepens your Ruby knowledge and shows you how it connects to Rails. You’ll gain confidence working with objects and classes and learn how to leverage Ruby’s elegant, expressive syntax for Rails application power. And you'll become a better Rails developer through a deep understanding of the design of Rails itself and how to take advantage of it.
Newcomers to Ruby will find a Rails-oriented Ruby introduction that’s easy to read and that includes dynamic programming techniques, an exploration of Ruby objects, classes, and data structures, and many neat examples of Ruby and Rails code in action.
Table Of Contents:
Part 1 The Ruby/Rails landscape 1
- 1 How Ruby works 3
- The mechanics of writing a Ruby program 4
- Getting the preliminaries in place 5, A Ruby literacy bootstrap guide 5, A brief introduction to method calls and Ruby objects, Writing and saving a sample program 8, Feeding the program to Ruby 9, Keyboard and file input 11, One program, multiple files 14
- Techniques of interpreter invocation 15
- Command-line switches 16, A closer look at interactive Ruby interpretation with irb 20
- Ruby extensions and programming libraries 21
- Using standard extensions and libraries 21, Using C extensions 22, Writing extensions and libraries 23
- Anatomy of the Ruby programming environment 24
- The layout of the Ruby source code 24, Navigating the Ruby installation 25, Important standard Ruby tools and applications 27
- Summary 31
- 2 How Rails works 33
- Inside the Rails framework 34
- A framework user’s–eye view of application development 35, Introducing the MVC framework concept 36, Meet MVC in the (virtual) flesh 37
- Analyzing Rails’ implementation of MVC 38
- A Rails application walk-through 41
- Introducing R4RMusic, the music-store application 42, Modeling the first iteration of the music-store domain 43, Identifying and programming the actions 50, Designing the views 53, Connecting to the application 58
- Tracing the lifecycle of a Rails run 59
- Stage 1: server to dispatcher 61, Stage 2: dispatcher, to controller 62, Stage 3: performance of a controller action 62, Stage 4: the fulfillment of the view 65
- Summary 65
- 3 Ruby-informed Rails development 67
- A first crack at knowing what your code does 69
- Seeing Rails as a domain-specific language 70, Writing program code with a configuration flavor 73, YAML and configuration that’s actually programming 75
- Starting to use Ruby to do more in your code 77
- Adding functionality to a controller 79, Deploying the Rails helper files 80, Adding functionality to models 82
- Accomplishing application-related skills and tasks 85
- Converting legacy data to ActiveRecord 85, The irb-based Rails application console 89
- Summary 90
Part 2 Ruby building blocks 93
- 4 Objects and variables 95
- From “things” to objects 96
- Introducing object-oriented programming 97, I, object! 98, Modeling objects more closely: the behavior of a ticket 103
- The innate behaviors of an object 108
- Identifying objects uniquely with the object_id method 109, Querying an object’s abilities with the respond_to? method 110, Sending messages to objects with the send method 111
- Required, optional, and default-valued arguments 112
- Required and optional arguments 112, Default values for arguments 113, Order of arguments 114
- Local variables and variable assignment 115
- Variable assignment in depth 117, Local variables and the things that look like them 119
- Summary 120
- 5 Organizing objects with classes 121
- Classes and instances 122
- A first class 123, Instance variables and object state 126
- Setter methods 130
- The equal sign (=) in method names 131, ActiveRecord properties and other =-method applications 133
- Attributes and the attr_* method family 136
- Automating the creation of attribute handlers 137, Two (getter/setter) for one 138, Summary of attr_* methods 139
- Class methods and the Class class 140
- Classes are objects too! 140, When, and why, to write a class method 141, Class methods vs. instance methods, clarified 143, The Class class and Class.new 144
- Constants up close 145
- Basic usage of constants 145, Reassigning vs. modifying constants 146
- Inheritance 148
- Inheritance and Rails engineering 149, Nature vs. nurture in Ruby objects 151
- Summary 153
- 6 Modules and program organization 154
- Basics of module creation and use 155
- A module encapsulating “stack-like-ness” 157, Mixing a module into a class 158, Leveraging the module further 160
- Modules, classes, and method lookup 163
- Illustrating the basics of method lookup 163, Defining the same method more than once 166, Going up the method search path with super 168
- Class/module design and naming 170
- Mix-ins and/or inheritance 171, Modular organization in Rails source and boilerplate code 173
- Summary 176
- 7 The default object (self) and scope 177
- Understanding self, the current/default object 179
- Who gets to be self, and where 179, Self as default receiver of messages 184, Instance variables and self 186
- Determining scope 188
- Global scope and global variables 188, Local scope 191, Scope and resolution of constants 194
- Deploying method access rules 197
- Private methods 197, Private methods as ActionController access protection 199, Protected methods 201
- Writing and using top-level methods 203
- Defining a top-level method 203, Predefined (built-in) top-level methods 204
- Summary 205
- 8 Control flow techniques 206
- Conditional code execution 207
- The if keyword and friends 208, Conditional modifiers 211, Case statements 211
- Repeating actions with loops 215
- Unconditional looping with the loop method 215, Conditional looping with the while and until keywords 216, Looping based on a list of values 218
- Code blocks, iterators, and the yield keyword 219
- The basics of yielding to a block 219, Performing multiple iterations 222, Using different code blocks 223, More about for 223
- Error handling and exceptions 225
- Raising and rescuing exceptions 225, Raising exceptions explicitly 227, Creating your own exception classes 228
- Summary 230
Part 3 Built-in classes and modules 231
- 9 Built-in essentials 233
- Ruby’s literal constructors 234
- Recurrent syntactic sugar 236
- Special treatment of += 237
- Methods that change their receivers (or don’t) 238
- Receiver-changing basics 239, bang (!) methods 240, Specialized and extended receiver-changing in ActiveRecord objects 241
- Built-in and custom to_* (conversion) methods 242
- Writing your own to_* methods 243
- Iterators reiterated 244
- Boolean states, Boolean objects, and nil 245
- True and false as states 246, true and false as objects 248, The special object nil 249
- Comparing two objects 251
- Equality tests 251, Comparisons and the Comparable module 252
- Listing an object’s methods 253
- Generating filtered and selective method lists 254
- Summary 255
- 10 Scalar objects 257
- Working with strings 258
- String basics 258, String operations 260, Comparing strings 265
- Symbols and their uses 267
- Key differences between symbols and strings 267, Rails-style method arguments, revisited 268
- Numerical objects 270
- Numerical classes 270, Performing arithmetic operations 271
- Times and dates 272
- Summary 275
- 11 Collections, containers, and enumerability 277
- Arrays and hashes compared 278
- Using arrays 279
- Creating a new array 279, Inserting, retrieving, and removing array elements 280, Combining arrays with other arrays 283, Array transformations 285, Array iteration, filtering, and querying 286, Ruby lessons from ActiveRecord collections 289
- Hashes 292
- Creating a new hash 293, Inserting, retrieving, and removing hash pairs 294, Combining hashes with other hashes 296, Hash transformations 297, Hash iteration, filtering, and querying 298, Hashes in Ruby and Rails method calls 301
- Collections central: the Enumerable module 303
- Gaining enumerability through each 304, Strings as Enumerables 306
- Sorting collections 307
- Sorting and the Comparable module 309, Defining sort order in a block 310
- Summary 311
- 12 Regular expressionsand regexp-basedstring operations 312
- What are regular expressions? 313
- A word to the regex-wise 314, A further word to everyone 314
- Writing regular expressions 314
- The regular expression literal constructor 315, Building a pattern 316
- More on matching and MatchData 319
- Capturing submatches with parentheses 319, Match success and failure 321
- Further regular expression techniques 323
- Quantifiers and greediness 323, Anchors and lookahead assertions 326, Modifiers 328, Converting strings and regular expressions to each other 329
- Common methods that use regular expressions 331
- String#scan 332, String#split 332, sub/sub! and gsub/gsub! 333, grep 334
- Summary 335
- 13 Ruby dynamics 337
- The position and role of singleton classes 338
- Where the singleton methods live 339, Examining and modifying a singleton class directly 340, Singleton classes on the method lookup path 342, Class methods in (even more) depth 345
- The eval family of methods 347
- eval 347, instance_eval 349, The most useful eval: class_eval (a.k.a. module_eval) 349
- Callable objects 351
- Proc objects 351, Creating anonymous functions with the lambda keyword 355, Code blocks, revisited 356, Methods as objects 357
- Callbacks and hooks 359
- Intercepting unrecognized messages with method_missing 360, Trapping include operations with Module#included 361, Intercepting inheritance with Class#inherited 363, Module#const_missing 365
- Overriding and adding to core functionality 365
- A cautionary tale 366
- Summary 367
Part 4 Rails through Ruby, Ruby throug Rails 369
- 14 (Re)modeling the R4RMusic application universe 371
- Tracking the capabilities of an ActiveRecord model instance 372
- An overview of model instance capabilities 373, Inherited and automatic ActiveRecord model behaviors 374, Semi-automatic behaviors via associations 378
- Advancing the domain model 380
- Abstracting and adding models (publisher and edition) 380, The instruments model and many-to-many relations 382, Modeling for use: customer and order 386
- Summary 390
- 15 Programmatically enhancing ActiveRecord models 392
- Soft vs. hard model enhancement 393
- An example of model-enhancement contrast 394
- Soft programmatic extension of models 396
- Honing the Work model through soft enhancements 398, Modeling the customer’s business 399, Fleshing out the Composer 401, Ruby vs. SQL in the development of soft enhancements 401
- Hard programmatic enhancement of model functionality 404
- Prettification of string properties 404, Calculating a work’s period 409, The remaining business of the Customer 414
- Extending model functionality with class methods 419
- Soft and hard class methods 419
- Summary 421
- 16 Enhancing the controllers and views 422
- Defining helper methods for view templates 424
- Organizing and accessing custom helper methods 425, The custom helper methods for R4RMusic 427
- Coding and deploying partial view templates 429
- Anatomy of a master template 429, Using partials in the welcome view template 430
- Updating the main controller 436
- The new face of the welcome action 436
- Incorporating customer signup and login 438
- The login and signup partial templates 438, Logging in and saving the session state 439, Gate-keeping the actions with before_filter 441, Implementing a signing-up facility 444, Scripting customer logout 445
- Processing customer orders 446
- The view_cart action and template 446, Viewing and buying an edition 448, Defining the add_to_cart action 449, Completing the order(s) 449
- Personalizing the page via dynamic code 450
- From rankings to favorites 450, The favorites feature in action 452
- Summary 454
- 17 Techniques for exploring the Rails source code 455
- Exploratory technique 1: panning for info 456
- Sample info panning: belongs_to 457
- Exploratory technique 2: shadowing Ruby 458
- Choosing a starting point 458, Choose among forks in the road intelligently 459, On the trail of belongs_to 460, A transliteration of belongs_to 463
- Exploratory technique 3: consulting the documentation 464
- A roadmap of the online Rails API documentation 466
- Summary 469
Appendix: Ruby and Rails installation and resources 471
index 477
Sample Chapters
Sample Chapter 5
Sample Chapter 10
The Amazon link is here
No comments:
Post a Comment